ajax概述
什么是异步,什么是同步?
假设有t1线程和t2线程,t1线程和t2线程并发,就是异步.假设在t2线程执行时,必须等待t1线程执行到某个位置之后t2才能执行,那么t2在等t1,排队的就是同步.ajax就是异步请求
XMLHttpRequest对象
XMLHttpRequest对象是ajax的核心对象,发送请求以及接受服务器数据的返回全靠这个对象.XMLhttpRequest对象,现代主流的浏览器都支持,都内置了改对象,可以直接使用.创建XMLHttpRequest对象
var xhr = new XMLHttpRequest();
|
XMLHttpRequest对象的方法
方法 |
描述 |
about() |
取消当前请求 |
getAllResponseHeaders() |
返回头部信息 |
getResponseHerader() |
返回特定的头部信息 |
open(method,url,async,user,psw) |
规定请求method:请求类型GET或者POST url:文件位置 ansync:true(异步)或者false(同步) user:可选的用户名称 psw:可选的密码 |
send() |
将请求返送到服务器,用于GET请求 |
send(string) |
将请求发送到服务器,用于POST请求 |
setRequestHeader() |
向要发送的报头添加标签/值对 |
XMLHttpRequest对象的属性
属性 |
描述 |
onreadystatechange |
定义当readyState属性发生变化时被调用的函数 |
readState |
保存XMLHttpRequest的状态. 0:表示未初始化 1:服务器连接已建立 2:请求已经收到 3:正在处理请求 4:请求已经完成并且响应就绪 |
responseText |
以字符串返回响应数据 |
responseXML |
以XML数据返回响应数据 |
status |
返回请求的状态号200:”OK” 403:”Forbidden” 404 :”Not Found” |
statusText |
返回状态文本(比如’OK’或者’Not Found’) |
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>发送ajax get请求</title> </head> <body>
<input type="button" value="hello ajax" id="helloBtn">
<div id="mydiv"></div>
<script type="text/javascript"> window.onload = function (){ document.getElementById("helloBtn").onclick = function (){ console.log("发送ajax get请求") } } </script> </body> </html>
|
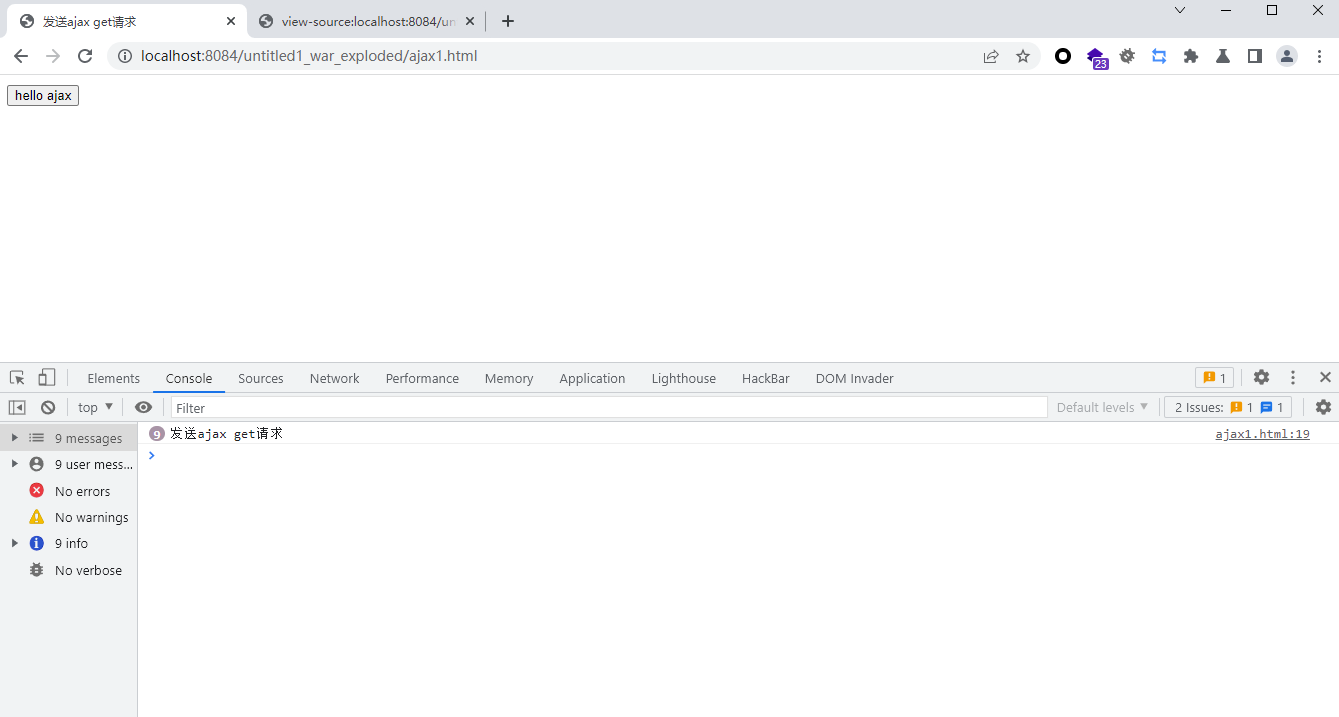
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>发送ajax get请求</title> </head> <body>
<input type="button" value="hello ajax" id="helloBtn">
<div id="mydiv"></div>
<script type="text/javascript"> window.onload = function (){ document.getElementById("helloBtn").onclick = function (){ var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function (){
if (this.readyState === 4){
if (this.status === 404){ alert("资源未找到") }else if (this.status === 500){ alert("服务器问题") }else if (this.status === 200){ alert("响应成功") alert(this.responseText) document.getElementById("mydiv").innerHTML = this.responseText } } } xhr.open("GET","/ajax/ajaxrequest1",true) xhr.send()
} } </script> </body> </html>
|
package wan.servlet;
import jakarta.servlet.ServletException; import jakarta.servlet.annotation.WebServlet; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse;
import javax.jws.WebService; import java.io.IOException; import java.io.PrintWriter;
@WebServlet("/ajaxrequest1") public class AjaxRequest1Servlet extends HttpServlet { @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException{
PrintWriter out = response.getWriter();
out.print("<font color='red'>welcome to ajax!!</font>");
} }
|
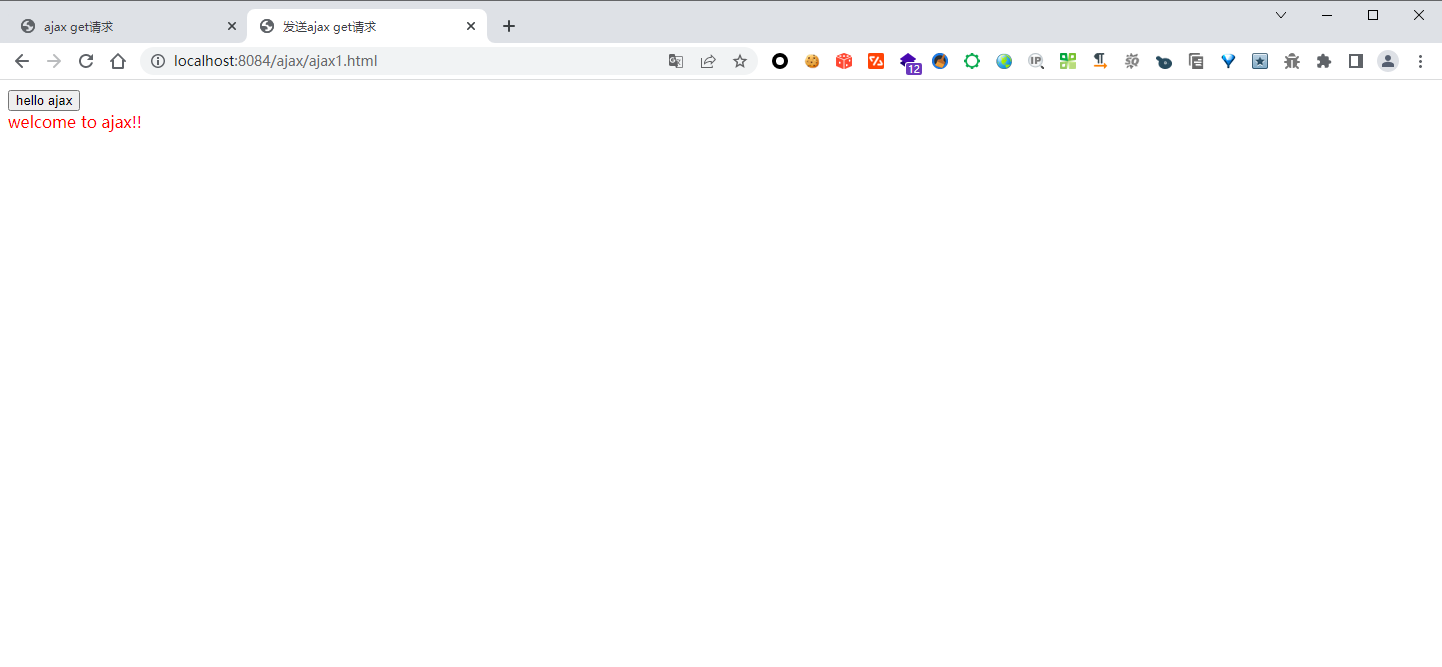
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>发送ajax get请求</title> </head> <body> usercode<input type="text" id="usercode"><br> username<input type="text" id="username"><br> <button id="btn">发送ajax get请求</button> <span id="myspan"></span> <div id="mydiv"></div>
<script type="text/javascript"> window.onload = function (){ document.getElementById("btn").onclick = function (){ var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function (){ if (this.readyState === 4 ){ if (this.status === 200){ document.getElementById("myspan").innerText = this.responseText }else { alert(this.status) } } }
var usercode = document.getElementById("usercode").value var username = document.getElementById("username").value alert(new Date().getTime()) xhr.open("GET","/ajax/ajaxrequest2?t="+Math.random()+"&usercode="+usercode+"&username="+username,true) xhr.send() } } </script>
</body> </html>
|
package wan.servlet;
import jakarta.servlet.ServletException; import jakarta.servlet.annotation.WebServlet; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse;
import javax.jws.WebService; import java.io.IOException; import java.io.PrintWriter;
@WebServlet("/ajaxrequest2") public class AjaxRequest2Servlet extends HttpServlet { @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException{
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
String usercode = request.getParameter("usercode"); String username = request.getParameter("username"); out.print("usercode" + usercode + ",username=" + username);
} }
|
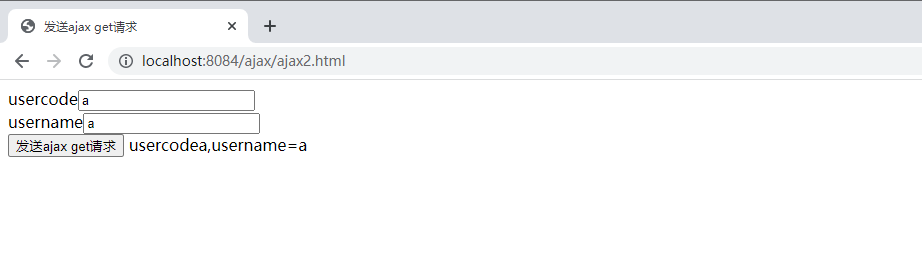
ajax发送post请求
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>发送ajax post请求</title> </head> <body> 用户名<input type="text" id="username"><br> 密码<input type="password" id="password"><br>
<button id="mybtn">发送ajax post请求</button> <div id="mydiv"></div>
<script type="text/javascript"> window.onload = function (){ document.getElementById("mybtn").onclick = function (){ var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function (){ if (this.readyState === 4){ if (this.status === 200){ document.getElementById("mydiv").innerHTML = this.responseText }else { alert(this.status) } } } xhr.open("POST","/ajax/ajaxrequest3",true) xhr.setRequestHeader("Content-Type","application/x-www-form-urlencoded")
var username = document.getElementById("username").value var password = document.getElementById("password").value xhr.send("username="+username+"&password="+password)
} } </script> </body> </html>
|
package wan.servlet;
import com.sun.deploy.net.HttpResponse; import jakarta.servlet.ServletException; import jakarta.servlet.annotation.WebServlet; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse;
import java.io.IOException; import java.io.PrintWriter;
@WebServlet("/ajaxrequest3") public class AjaxRequest3Servlet extends HttpServlet { @Override protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException{ response.setContentType("text/html;charset=UTF-8"); PrintWriter out = response.getWriter();
String username = request.getParameter("username"); String password = request.getParameter("password"); out.print("用户名是:" + username+"密码是:"+password); } }
|
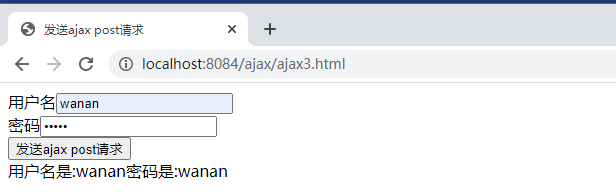
java的数据库连接
下载mysql驱动
https://cdn.mysql.com//Downloads/Connector-J/mysql-connector-java-8.0.29.zip
要把jar包放到WEB-INF目录之下
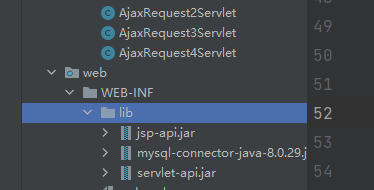
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>ajax post验证用户名是否可用</title> </head> <body> 用户名:<input type="text" id="username"> <span id="tipMsg">
</span>
<script type="text/javascript"> window.onload = function (){ document.getElementById("username").onfocus = function (){ document.getElementById("tipMsg").innerHTML = ""
} document.getElementById("username").onblur = function (){ var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function () { if (this.readyState === 4){ if (this.status === 200 ){ document.getElementById("tipMsg").innerHTML = this.responseText }else { alert(this.status) } } } xhr.open("POST","/ajax/ajaxrequest4",true) xhr.setRequestHeader("Content-Type","application/x-www-form-urlencoded") var username = document.getElementById("username").value xhr.send("uname="+username) } } </script>
</body> </html>
|
package wan.servlet;
import com.sun.deploy.net.HttpResponse; import jakarta.servlet.ServletException; import jakarta.servlet.annotation.WebServlet; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse;
import java.io.IOException; import java.io.PrintWriter; import java.sql.*;
@WebServlet("/ajaxrequest4") public class AjaxRequest4Servlet extends HttpServlet { @Override protected void doPost(HttpServletRequest request, HttpServletResponse response)throws ServletException, IOException{ String uname =request.getParameter("uname");
boolean flag = false;
Connection conn = null; PreparedStatement ps = null; ResultSet rs = null;
try {
Class.forName("com.mysql.cj.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/security?useUnicode=true&characterEncoding=utf8&serverTimezone=UTC","root","wananw");
String sql = "select id,username from users where username = ?";
ps = conn.prepareStatement(sql);
ps.setString(1,uname);
rs = ps.executeQuery(); if (rs.next()){ flag = true;
}
} catch (Exception e) { e.printStackTrace(); }finally {
if (rs != null){ try { rs.close(); } catch (SQLException e) { e.printStackTrace(); } } if (ps != null){ try { ps.close(); } catch (SQLException e) { e.printStackTrace(); } } if (conn != null){ try { conn.close(); } catch (SQLException e) { e.printStackTrace(); } }
}
response.setContentType("text/html;charset=UTF-8"); PrintWriter out = response.getWriter(); if (flag){
out.print("<font color='red'>对不起,用户名已存在</font>"); }else{ out.print("<font color='green'>用户名可以使用</font>"); }
} }
|
ajax加json返回信息
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>发送ajax请求 显示学生列表</title> </head> <body> <script type="text/javascript"> window.onload = function (){ document.getElementById("btn").onclick = function (){ var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function () { if (this.readyState === 4){ if (this.status === 200){ var stuList = JSON.parse(this.responseText) var html = "" for (var i = 0; i < stuList.length;i++){ var stu = stuList[i] html += "<tr>" html += "<td>"+(i+1)+"</td>" html += "<td>"+stu.name+"</td>" html += "<td>"+stu.age+"</td>" html += "<td>"+stu.addr+"</td>" html += "</tr>" } document.getElementById("stutbody").innerHTML = html
}else { alert(this.status)
} } } xhr.open("GET","/ajax/ajaxrequest5?t=" + new Date().getTime(),true) xhr.send() } } </script> <input type="button" value="显示学生列表" id="btn"> <table width="50%" border="1px"> <thead> <tr> <th>序号</th> <th>姓名</th> <th>年龄</th> <th>住址</th> </tr>
</thead> <tbody id="stutbody">
</tbody> </table>
</body> </html>
|
package wan.servlet;
import jakarta.servlet.ServletException; import jakarta.servlet.annotation.WebServlet; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse;
import java.io.IOException; import java.io.PrintWriter;
@WebServlet("/ajaxrequest5") public class AjaxRequest5Servlet extends HttpServlet { @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException , IOException{
response.setContentType("text/html;charset=UTF-8"); PrintWriter out = response.getWriter();
StringBuilder html = new StringBuilder(); html.append("<tr>"); html.append("<td>1</td>"); html.append("<td>王五</td>"); html.append("<td>20</td>"); html.append("<td>北京大兴区</td>"); html.append("</tr>"); html.append("<tr>"); html.append("<td>2</td>"); html.append("<td>李四</td>"); html.append("<td>22</td>"); html.append("<td>北京海淀区</td>"); html.append("</tr>");
out.print(html);
} }
|
json
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>json test</title> </head> <body> <script type="text/javascript">
var user = { "usercode" : 111, "username" : "zhangsan", "sex" : true, "age" : 20, "aihaos" : ["抽烟", "喝酒", "烫头"], "addr" : { "city" : "北京", "street" : "北京大兴区", "zipcode" : "123456" } } console.log(user.usercode) console.log(user.username) console.log(user.sex ? "男":"女") console.log(user.age)
for (var i = 0 ; i < user.aihaos.length;i++){ console.log(user.aihaos[i]) }
console.log(user.addr.street)
console.log(user["usercode"]) console.log(user["username"]) console.log(user["sex"]?"男":"女") console.log(user["age"])
</script>
</body> </html>
|
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>json </title> </head> <body>
<script type="text/javascript"> var user = { "username" : "zhangsan", "password" : "123456" }
alert(user.username+","+user.password)
var fromJavaServerJsonStr = "{\"usercode\" : 111, \"age\" : 20}"
var jsonobj = JSON.parse(fromJavaServerJsonStr) alert(jsonobj.usercode +"," + jsonobj.password) </script> </body> </html>
|
package wan.servlet;
import jakarta.servlet.ServletException; import jakarta.servlet.annotation.WebServlet; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse;
import java.io.IOException; import java.io.PrintWriter;
@WebServlet("/ajaxrequest5") public class AjaxRequest5Servlet extends HttpServlet { @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException , IOException{
response.setContentType("text/html;charset=UTF-8"); PrintWriter out = response.getWriter();
String jsonStr = "[{\"name\":\"王五\",\"age\":20,\"addr\":\"北京大兴区\"}, {\"name\":\"李四\",\"age\":22,\"addr\":\"北京海淀区\"}]"; out.print(jsonStr); } }
|
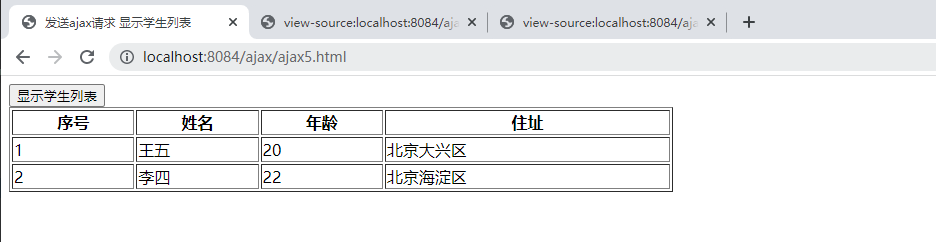
package wan.servlet;
import jakarta.servlet.ServletException; import jakarta.servlet.annotation.WebServlet; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse;
import java.awt.dnd.DragGestureEvent; import java.io.IOException; import java.io.PrintWriter; import java.sql.*;
@WebServlet("/ajaxrequest5") public class AjaxRequest5Servlet extends HttpServlet { @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException , IOException{
response.setContentType("text/html;charset=UTF-8"); PrintWriter out = response.getWriter();
StringBuilder json = new StringBuilder(); String jsonStr = "";
Connection coon = null; PreparedStatement ps = null; ResultSet rs = null; try { Class.forName("com.mysql.jdbc.Driver"); coon = DriverManager.getConnection("jdbc:mysql://localhost:3306/security?useUnicode=true&characterEncoding=utf8&serverTimezone=UTC","root","wananw"); String sql = "select id,username,password from users"; ps = coon.prepareStatement(sql); rs = ps.executeQuery();
json.append("["); while (rs.next()) { String name = rs.getString("id"); String age = rs.getString("username"); String addr = rs.getString("password"); json.append("{\"name\":\""); json.append(name); json.append("\",\"age\":\""); json.append(age); json.append("\",\"addr\":\""); json.append(addr); json.append("\"},"); } jsonStr = json.substring(0, json.length() - 1) + "]";
} catch (Exception e) { e.printStackTrace(); }finally { if (rs !=null){ try { rs.close(); } catch (SQLException e) { e.printStackTrace(); }
} if (ps != null){ try { ps.close(); } catch (SQLException e) { e.printStackTrace();
} } if (coon!= null){ try { coon.close(); } catch (SQLException e) { e.printStackTrace(); } } }
out.print(jsonStr); } }
|
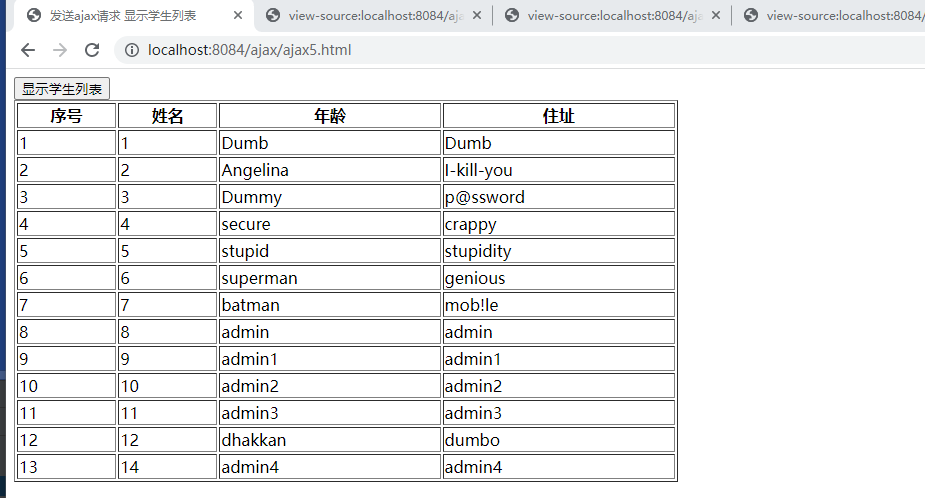
fastjson使用
User.java
package wan.servlet;
public class User { private String id; private String username; private int age;
public User() { }
public String getId() { return id; }
public void setId(String id) { this.id = id; }
public String getUsername() { return username; }
public void setUsername(String username) { this.username = username; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
public User(String id, String username, int age) { this.id = id; this.username = username; this.age = age; } }
|
Fastjsion.java
package wan.servlet;
import com.alibaba.fastjson.JSON;
import java.util.ArrayList; import java.util.List;
public class Fastjson { public static void main(String[] args) { User user = new User("111","zhangsan",20);
String jsonStr = JSON.toJSONString(user);
System.out.print(jsonStr);
List<User> userList = new ArrayList<>(); User user1 = new User("120", "lisi", 30); User user2 = new User("130", "jackson", 33); userList.add(user1); userList.add(user2); String jsonStr2 = JSON.toJSONString(userList); System.out.print(jsonStr2); } }
|

package wan.servlet;
import com.alibaba.fastjson.JSON; import jakarta.servlet.ServletException; import jakarta.servlet.annotation.WebServlet; import jakarta.servlet.http.HttpServlet; import jakarta.servlet.http.HttpServletRequest; import jakarta.servlet.http.HttpServletResponse;
import java.awt.dnd.DragGestureEvent; import java.io.IOException; import java.io.PrintWriter; import java.sql.*; import java.util.ArrayList; import java.util.List;
@WebServlet("/ajaxrequest5") public class AjaxRequest5Servlet extends HttpServlet { @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException , IOException{
response.setContentType("text/html;charset=UTF-8"); PrintWriter out = response.getWriter();
StringBuilder json = new StringBuilder(); String jsonStr = "";
Connection coon = null; PreparedStatement ps = null; ResultSet rs = null; try { Class.forName("com.mysql.jdbc.Driver"); coon = DriverManager.getConnection("jdbc:mysql://localhost:3306/security?useUnicode=true&characterEncoding=utf8&serverTimezone=UTC","root","wananw"); String sql = "select id,username,password from users"; ps = coon.prepareStatement(sql); rs = ps.executeQuery();
List<Student> studentList = new ArrayList<>(); while (rs.next()){ int id = rs.getInt("id"); String username = rs.getString("username"); String password = rs.getString("password");
Student s = new Student(id,username,password);
studentList.add(s); } jsonStr = JSON.toJSONString(studentList); } catch (Exception e) { e.printStackTrace(); }finally { if (rs !=null){ try { rs.close(); } catch (SQLException e) { e.printStackTrace(); }
} if (ps != null){ try { ps.close(); } catch (SQLException e) { e.printStackTrace();
} } if (coon!= null){ try { coon.close(); } catch (SQLException e) { e.printStackTrace(); } } }
out.print(jsonStr); } }
|
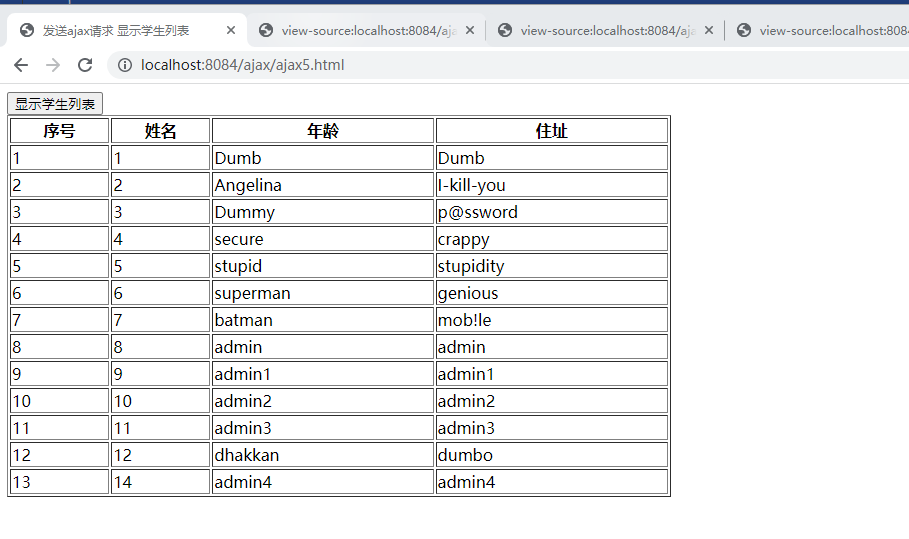